Git Commit Message Guidelines
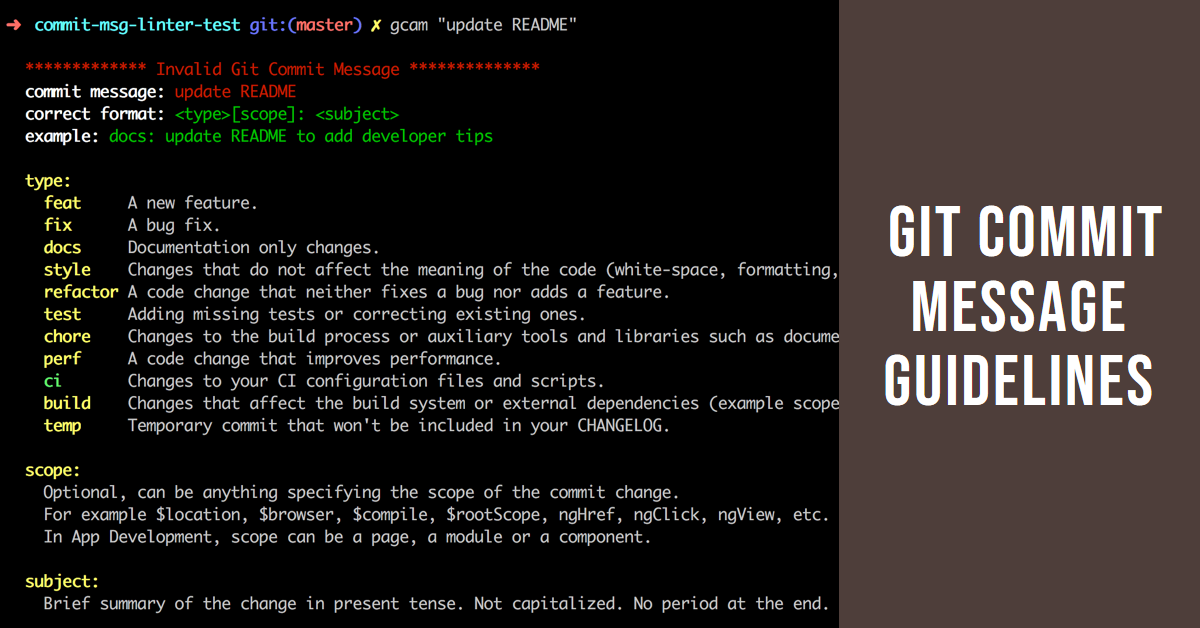
Git commit message conventions help maintain consistency and clarity in the development process. When multiple developers are working on a project, it’s important to have a common format for commit messages so that everyone can easily understand what changes were made, why they were made, and how significant those changes are. These conventions make it easier to navigate through the history of commits, identify issues or bugs, and review code.
Goals
Automatically Generating CHANGELOG.md
Use the following three sections in changelog: new features
, bug fixes
, breaking changes
.
This list could be generated by script when doing a release. Along with links to related commits.
Of course you can edit this change log before actual release, but it could generate the skeleton.
List of all subjects (first lines in commit message) since last release:
|
|
New features in this release
|
|
Recognizing Unimportant Commits
These are formatting changes (adding/removing spaces/empty lines, indentation), missing semi colons, comments. So when you are looking for some change, you can ignore these commits - no logic change inside this commit.
When bisecting, you can ignore these by:
|
|
Improved Code Quality
Well-written commit messages help developers understand the purpose and context of code changes, leading to improved code quality. Clear and concise messages facilitate code reviews and help identify potential issues early on.
Easier Collaboration
When team members follow consistent commit message conventions, it becomes easier to collaborate on projects. Everyone can quickly grasp the intent behind the changes, reducing confusion and miscommunication. This promotes smoother collaboration and efficient code merging.
Enhanced Code History
Commit messages create a detailed history of code changes, providing valuable insights into the evolution of the project. Standardized messages make it easier to track the progress of specific features or bug fixes, aiding in debugging, refactoring, and understanding the rationale behind past decisions.
Facilitated Project Management
Clear commit messages make it easier for project managers to monitor the progress of tasks and identify dependencies. Well-structured messages align with agile methodologies and improve overall project transparency.
Improved Documentation
Commit messages act as documentation for code changes. They provide a concise summary of the modifications, capturing the essence of the changes in a human-readable format. This documentation aids in understanding the codebase, particularly for newcomers or team members working on unfamiliar parts of the project.
Automation and Tool Integration
Standardized commit messages facilitate the integration of tools and automation scripts. For instance, some tools can automatically generate changelogs, release notes, or documentation based on the commit messages. Consistent formatting allows for better parsing and processing of commit messages.
Version Control History
Commit messages provide a chronological record of code changes, allowing developers to easily revert to previous versions of the codebase if necessary. Having informative messages makes it easier to identify specific commits and understand the state of the code at different points in time.
Enhanced Search and Traceability
Standardized commit messages improve the searchability of code changes within version control systems. Developers can quickly find commits related to specific keywords, making it easier to trace the history of a particular feature or bug. This enables faster problem-solving and facilitates continuous improvement.
Professionalism and Team Culture
Employing consistent commit message conventions reflects a professional and organized approach to software development. It demonstrates a commitment to maintain a high-quality codebase and fosters a culture of collaboration and transparency within the development team.
Commit Message Format
Each commit message consists of a header
, a body
and a footer
. The header has a special format that includes a type, a scope and a subject:
|
|
The header
is mandatory and the scope
of the header is optional.
Any line of the commit message cannot be longer 100 characters! This allows the message to be easier to read on GitHub as well as in various git tools.
The footer should contain a closing reference to an issue if any.
Header
The message header is a single line that contains succinct description of the change containing a type, an optional scope and a subject.
Type
Must be one of the following:
- build: Changes that affect the build system or external dependencies (example scopes: gulp, broccoli, npm)
- ci: Changes to CI configuration files and scripts (example scopes: Travis, Circle, BrowserStack, SauceLabs)
- docs: Documentation only changes
- feat: A new feature
- fix: A bug fix
- perf: A code change that improves performance
- refactor: A code change that neither fixes a bug nor adds a feature
- style: Changes that do not affect the meaning of the code (white-space, formatting, missing semi-colons, etc)
- test: Adding missing tests or correcting existing tests
Scope
Scope is used to describe the scope of influence of commit. For example api, model, compile, etc…
You can use *
if there isn’t a more fitting scope.
Subject
The subject contains a succinct description of the change:
- use the imperative, present tense: “change” not “changed” nor “changes”
- don’t capitalize the first letter
- no dot (.) at the end
Body
Just as in the subject, use the imperative, present tense: “change” not “changed” nor “changes”. The body should include the motivation for the change and contrast this with previous behavior.
Footer
The footer should contain any information about Breaking Changes and is also the place to reference issues that this commit Closes.
Breaking Changes
All breaking changes have to be mentioned as a breaking change block in the footer, which should start with the word BREAKING CHANGE:
with a space or two newlines. The rest of the commit message is then the description of the change, justification and migration notes.
|
|
Referencing Issues
Closed bugs should be listed on a separate line in the footer prefixed with “Closes” keyword like this:
Closes #234
or in case of multiple issues:
Closes #123, #245, #992
Revert
If the commit reverts a previous commit, its header should begin with revert:
, followed by the header of the reverted commit. In the body it should say: This reverts commit <hash>.
, where the hash is the SHA of the commit being reverted.
Examples
feat($browser): onUrlChange event (popstate/hashchange/polling)
Added new event to $browser:
- forward popstate event if available
- forward hashchange event if popstate not available
- do polling when neither popstate nor hashchange available
Breaks $browser.onHashChange, which was removed (use onUrlChange instead)
fix($compile): couple of unit tests for IE9
Older IEs serialize html uppercased, but IE9 does not… Would be better to expect case insensitive, unfortunately jasmine does not allow to user regexps for throw expectations.
Closes #392 Breaks foo.bar api, foo.baz should be used instead
feat(directive): ng:disabled, ng:checked, ng:multiple, ng:readonly, ng:selected
New directives for proper binding these attributes in older browsers (IE). Added coresponding description, live examples and e2e tests.
Closes #351
style($location): add couple of missing semi colons
docs(guide): updated fixed docs from Google Docs
Couple of typos fixed:
- indentation
- batchLogbatchLog -> batchLog
- start periodic checking
- missing brace
Linter Tools
- git-commit-msg-linter: A lightweight, independent, 0 configurations and joyful git commit message linter.
- husky: You can use it to lint your commit messages, run tests, lint code, etc… when you commit or push. Husky supports all client-side Git hooks.