Git Submodule: Mastering Modular Development in Git
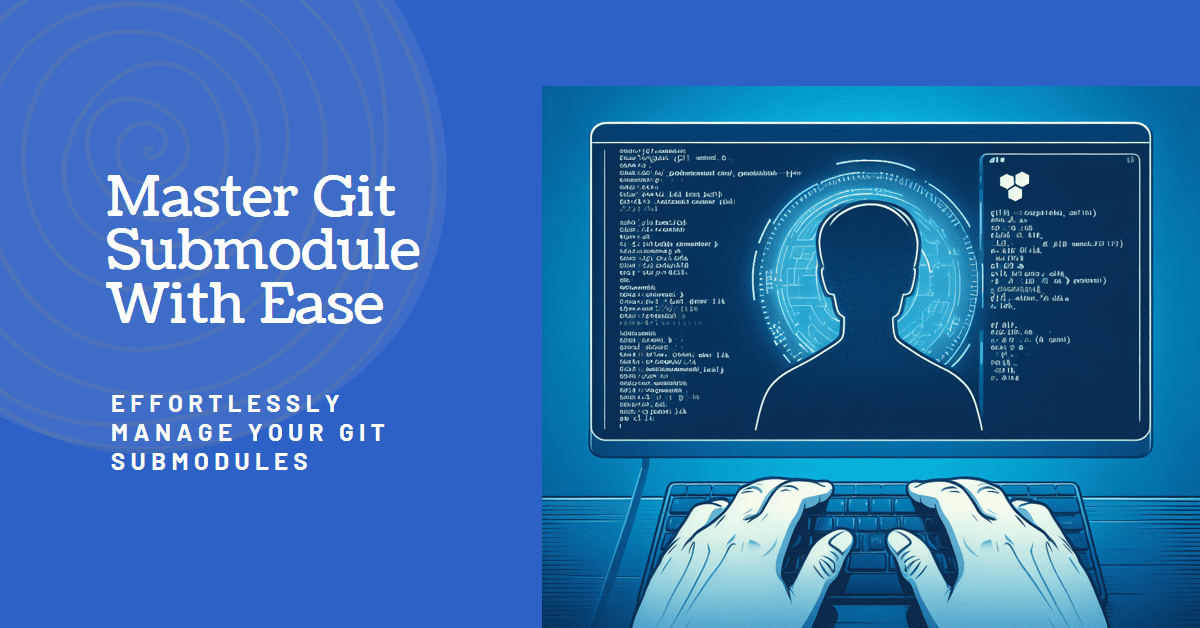
Introduction
Git submodule is a powerful feature that allows you to include other Git repositories as part of your project. This can be incredibly useful for managing external dependencies or shared components across multiple projects.
Benefits of Using Git Submodules
- Modular Development: Break large projects into smaller, independent modules, each maintained in its own Git repository.
- Code Reusability: Share code among different projects by referencing a submodule instead of duplicating the code.
- Collaboration: Enable multiple teams to work on different modules concurrently.
- Dependency Management: Keep track of and update dependencies as part of your project’s version history.
Drawbacks of Using Git Submodules
- Complexity: Managing submodules can add complexity to your project structure and history.
- Updates: Submodule updates can be more involved than updating regular files.
- Dependencies: Submodules introduce external dependencies, which can impact the stability and maintenance of your project.
When to Use Submodules
- External dependencies with specific version control: Ensure consistent integration of external libraries or components that you don’t directly control, especially when specific versions are required.
- Independently developed subprojects: Manage multiple repositories within a larger project, allowing teams to work autonomously on different parts.
- Tracking vendor code: Track external code that isn’t frequently updated, maintaining a specific version for stability.
Adding a Submodule
To add a submodule to your project, you first need to initialize a Git repository in the directory where you want the submodule to live. Once you have done that, you can use the git submodule add
command to add the submodule. The syntax for this command is:
|
|
- This command adds the specified Git repository as a submodule in the directory
<submodule-directory>
within your current repository.
Updating a Submodule
- Fetch latest changes from the submodule’s remote repository:
|
|
- Update the actual content of the submodule:
|
|
- Optionally, specify the submodule path:
|
|
Committing Submodule Changes
When you make changes to a submodule, you can commit those changes to your local repository using the git add
and git commit
commands. Once you have committed your changes, you can push them to the remote repository using the git push
command.
Removing a Submodule
- To remove a submodule from your project, use:
|
|
- This will remove the submodule from your Git history and from your working tree.
Key Practices
1. Use for external dependencies, not internal code
Submodules are best suited for external code. For internal code, consider alternative approaches like splitting into separate repositories or using Git subtrees.
2. Avoid deep nesting
Excessive nesting can complicate management. Flatten the structure if possible.
3. Initialize and update recursively
Clone with git clone --recursive
to fetch submodules automatically. Update them with git submodule update --init --recursive
.
4. Commit changes within submodules
Make changes within the submodule’s directory and commit them there. Then commit the updated reference in the parent repository.
5. Keep .gitmodules file updated
This file tracks submodule information. Ensure it reflects the correct URLs and paths.
6. Sync submodules before operations
Use git submodule sync
to ensure local configuration matches remote settings.
7. Communicate changes effectively
Inform team members about submodule updates or modifications to avoid conflicts.
8. Consider alternatives for frequent updates
If a submodule requires constant updates, consider using Git subtrees for easier integration.
9. Automate tasks
Use scripts or tools to automate common submodule operations, reducing manual effort and potential errors.
10. Document usage
Clearly document submodule usage, including their purpose, expected behavior, and update procedures.
Tips
- Prefer shallow cloning for submodules to minimize download size.
- Use branches within submodules to manage different versions or features.
- Consider using tools like
git submodule foreach
to run commands across all submodules.
Remember: Submodules can be powerful for managing dependencies, but they require careful handling to avoid complexities. Adhere to these best practices to ensure effective usage and maintain project consistency.
Conclusion
Git submodules offer a powerful way to incorporate modular development and code reusability into your projects. While they can introduce some complexity, they can streamline collaboration, dependency management, and version tracking. Understanding the benefits and drawbacks of using submodules will help you make informed decisions about their use in your projects.