Demystifying JWT
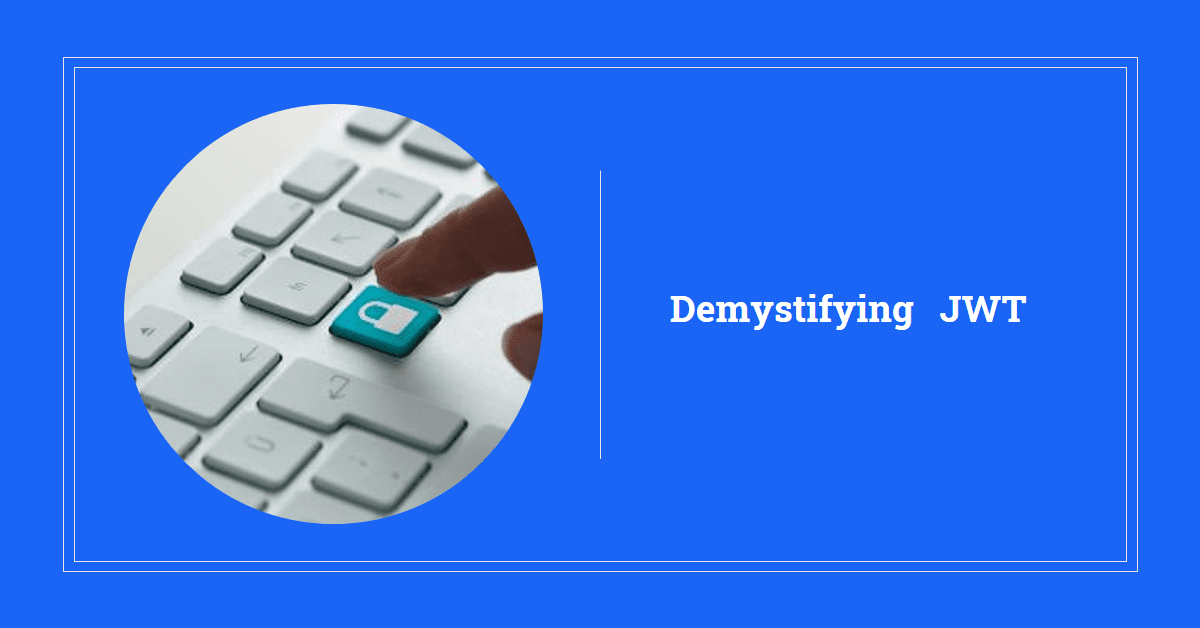
JSON Web Token (JWT) are a popular choice for securing APIs and web applications. However, they’re often misunderstood or misused. In this post, we’ll demystify JWTs and provide a clear understanding of how they work and when to use them.
What is a JWT?
A JWT is a JSON object that contains a header
, a payload
, and a signature
. The header typically includes information about the type of token being used, while the payload contains the data that’s being transmitted. The signature is generated using a secret key and ensures the integrity and authenticity of the token.
JWT Structure
Here’s an example of a JWT structure:
|
|
In this example, the header specifies that the token is a Bearer token using the HS256 algorithm for signing. The payload contains the user’s ID, name, and email address. The signature is generated using a secret key and is used to verify the authenticity of the token.
How JWTs Work
Here’s how JWTs work:
- Token Generation: When a user requests access to a protected resource, the server generates a JWT using a secret key. The token includes the user’s ID and any other relevant information.
- Token Signing: The server signs the JWT using a digital signature algorithm, such as HS256 or RSA. This ensures that the token cannot be tampered with or altered in transit.
- Token Transmission: The server sends the JWT to the client, who then stores it locally.
- Token Verification: When the client makes a subsequent request to a protected resource, they include the JWT in the
Authorization
header. The server verifies the token’s digital signature and ensures that it has not been tampered with. If the token is valid, the server grants access to the requested resource.
Advantages of JWTs
JWTs have several advantages over other authentication mechanisms:
- Stateless: JWTs do not require a server-side session store, making them suitable for use in stateless applications.
- Compact: JWTs are compact and can be easily stored in a small amount of space, making them ideal for use in mobile apps or other bandwidth-constrained clients.
- Secure: JWTs use digital signatures to ensure their integrity and authenticity, making them secure against tampering and eavesdropping attacks.
- Customizable: JWTs can be customized to include any data that’s relevant to the application, such as user IDs, names, or permissions.
- Long-lived: JWTs can be set to expire after a certain time, but they can also be refreshed to extend their lifetime, making them suitable for long-term authentication.
Disadvantages of JWTs
While JWTs have many advantages, they also have some disadvantages:
- Security Risks: If the secret key used to sign the JWT is compromised, an attacker can generate valid tokens and gain unauthorized access to protected resources. Therefore, it’s essential to keep the secret key secure and use appropriate security measures, such as rotating keys regularly.
- Token Forgery: An attacker could potentially forge a JWT and impersonate a legitimate user. To mitigate this risk, it’s important to ensure that the client is unable to modify the token in any way.
- Token Lifecycle Management: JWTs have a limited lifetime, and managing their lifecycle can be complex, especially in scenarios where tokens need to be revoked or updated.
When to Use JWTs
JWTs are suitable for use cases where stateless, compact, and secure authentication is required. Examples include:
- API Authentication: JWTs are commonly used to secure RESTful APIs and protect them against unauthorized access.
- Web Application Security: JWTs can be used to secure web applications and provide long-term authentication for users.
- Mobile Application Security: JWTs are suitable for use in mobile apps, where they can provide secure authentication and authorization without requiring a server-side session store.
Conclusion
JSON Web Tokens (JWT) are a powerful tool for securing APIs and web applications. They’re stateless, compact, secure, customizable, and long-lived, making them suitable for a wide range of use cases. However, they also have some disadvantages, such as security risks and token forgery. By understanding how JWTs work and when to use them, you can ensure that your application is secure and well-protected against unauthorized access.