Microservices Design Patterns
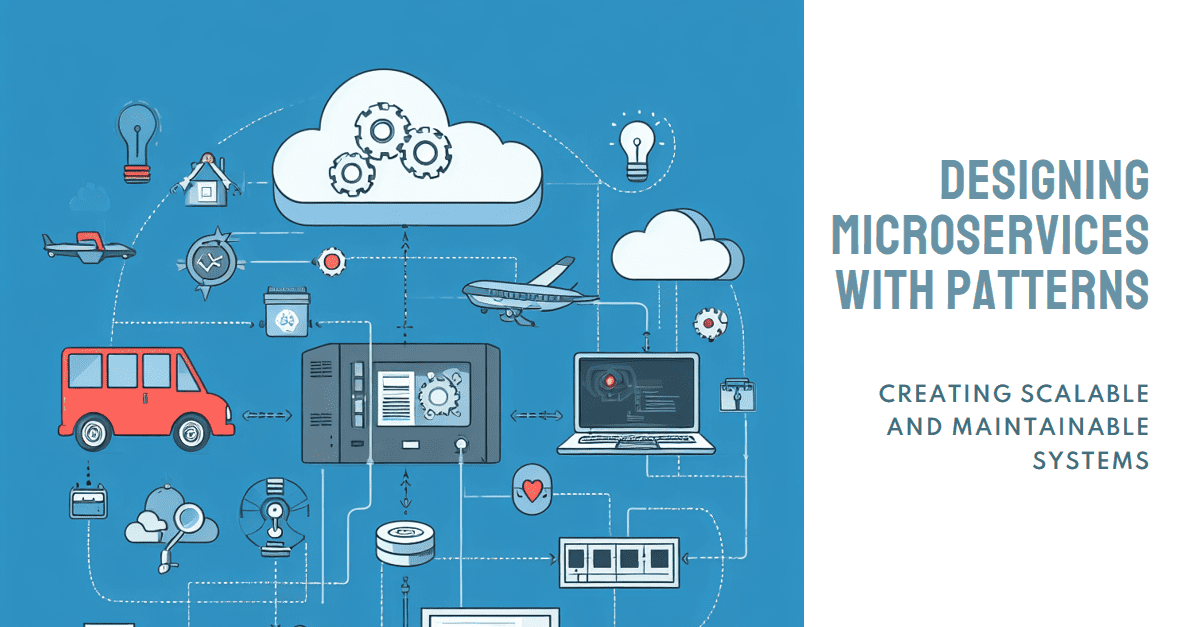
Microservices architecture has become a popular approach to software development in recent years, and with good reason. By breaking down a large monolithic application into smaller, independent services, teams can develop, test, and deploy independently, leading to faster time-to-market and increased scalability. However, designing a microservices architecture can be challenging, especially when it comes to dealing with the complexities of distributed systems. That’s where design patterns come in.
What Are Microservices?
Microservices are a modern approach to software development where an application is composed of a collection of loosely coupled services. Each service is responsible for a single, well-defined task and communicates with other services through lightweight protocols. Microservices can be deployed independently, scaled individually, and developed by different teams.
Benefits of Microservices
Modularity
Microservices are designed to be modular, allowing teams to work independently on different parts of the application. This can speed up development and make it easier to maintain the application over time.
Scalability
Microservices allow for better scalability compared to monolithic architecture. Since each service is independent, it can be scaled up or down without affecting the entire application. This means that resources can be allocated more efficiently and bottlenecks can be resolved more quickly.
Flexibility
Microservices offer greater flexibility in terms of technology and language choice. Each service can be developed using a different programming language, framework, or database, allowing developers to choose the best tools for the specific task at hand.
Resilience
With microservices, if one service fails, it won’t bring down the entire application. This increases the overall resilience of the system and reduces the risk of a single point of failure.
Agility
Microservices can be deployed and updated more frequently than traditional monolithic applications. This can help to improve the speed at which new features are released and reduce the risk of downtime.
Faster Development
Microservices allow for faster development cycles since each service can be developed independently. This means that new features or functionality can be added to the application more quickly, allowing for faster time-to-market.
Drawbacks of Microservices
Increased Complexity
Microservices introduce additional complexity compared to monolithic architecture. This includes the need to manage multiple services, ensure communication and data consistency between services, and handle failures and errors.
Higher Operational Overhead
Microservices require more effort to deploy, manage, and maintain compared to monolithic architecture. This includes tasks such as service discovery, load balancing, and monitoring.
Network Latency
Since microservices are distributed, network latency can become a concern. This can impact the performance of the application and require additional effort to mitigate.
Data Consistency
Microservices introduce additional complexity when it comes to data consistency. Since each service has its own database, ensuring data consistency across services can be challenging.
- Complexity: Microservices can be more complex to design and implement than traditional monolithic applications. This can lead to increased development time and costs.
- Communication overhead: The communication between microservices can be a source of overhead, which can impact performance.
- Testing: Testing microservices can be more difficult than testing monolithic applications. This can lead to increased testing time and costs.
When to Use Microservices
Microservices are a good choice for applications that are:
- Complex and require a high degree of modularity.
- Scalable and need to be able to handle a high volume of traffic.
- Resilient and need to be able to tolerate failures.
- Agile and need to be able to be deployed and updated frequently.
Inapplicable Scenarios
Microservices may not be suitable for simple, small-scale applications that do not require a high degree of scalability or flexibility. They may also not be appropriate for applications that require a high level of data consistency or transactions across multiple services.
Microservices Design Patterns
API Gateway Pattern
The API Gateway is the single entry point for all client requests to a microservices-based application. It decouples the clients from the individual microservices and provides a unified interface for accessing the application’s functionality.
Benefits of API Gateway
- Centralized control and security: The API gateway acts as a central point for enforcing security policies, authentication, and authorization. It can also handle tasks such as rate limiting, load balancing, and monitoring.
- Improved performance: The API gateway can cache frequently requested data and perform optimizations to improve the performance of the application.
- Simplified development: By providing a single entry point for clients, the API gateway can simplify the development of new microservices. Developers can focus on building the business logic of their microservices without worrying about the details of how they will be accessed by clients.
Implementation
The API gateway can be implemented using a variety of technologies, such as Nginx, Apache Traffic Server, or Kong. It is typically deployed as a reverse proxy in front of the microservices. When a client makes a request to the API gateway, the gateway routes the request to the appropriate microservice.
Variations
There are a number of variations of the API gateway pattern, including:
- Service mesh: A service mesh is a dedicated infrastructure layer that provides connectivity, load balancing, service discovery, and other features for microservices. Service meshes typically include an API gateway as a component.
- API management platform: An API management platform is a software platform that provides a set of tools for managing APIs, including API gateways, developer portals, and analytics dashboards.
- Reverse proxy: A reverse proxy is a server that sits in front of a group of servers and forwards client requests to the appropriate server. API gateways can be implemented using reverse proxies.
Service Registry Pattern
This pattern involves maintaining a central registry of services that are available in the system. The registry contains information about each service’s location, metadata, and status. Services can register themselves with the registry when they start up, and the registry can be used to route requests between services. The pattern aims to simplify the process of locating and communicating with multiple microservices in a distributed system.
Key Concepts
Service Discovery
The Service Registry serves as a central hub for registering and discovering microservices. Each microservice registers its location and information with the registry upon startup. When a consumer needs to access a particular service, it queries the registry to obtain the necessary details for establishing a connection.
Load Balancing
The Service Registry enables load balancing by providing a mechanism to distribute traffic across multiple instances of a microservice. This helps ensure that individual instances are not overloaded, improving overall system performance and availability. The registry may employ various algorithms to distribute requests efficiently.
Health Checking
To ensure the reliability and availability of microservices, the Service Registry often includes health checking mechanisms. It periodically checks the status of registered services to identify any failures or degradations. If a service is found to be unhealthy, its information may be temporarily removed from the registry or marked as such, preventing consumers from attempting to connect to unavailable services.
Service Update Notification
The Service Registry provides a way to notify consumers when a service’s information changes. This is useful for handling scenarios such as service updates, migrations, or scaling events. By receiving notifications, consumers can adapt to changes and seamlessly maintain connections with the updated services.
Benefits of Service Registry Pattern
-
Centralized Service Management: The Service Registry provides a single point of control for managing and monitoring microservices. This simplifies the process of adding, removing, or updating services, as well as tracking their health and performance.
-
Dynamic Discovery and Load Balancing: The Service Registry enables dynamic service discovery, allowing consumers to locate services automatically without hard-coded dependencies. Load balancing is also simplified, as the registry helps distribute requests across available instances efficiently.
-
Loose Coupling: The Service Registry promotes loose coupling between microservices by abstracting the discovery and communication mechanisms. This allows services to be developed and deployed independently, reducing dependencies and enhancing flexibility.
-
Scalability and Resilience: The Service Registry facilitates scalability by supporting multiple instances of microservices. It also contributes to resilience by providing mechanisms for health checking and service updates, ensuring that consumers can access available and healthy services.
Circuit Breaker Pattern
The Circuit Breaker pattern is a resilience mechanism used in microservices design patterns to handle network and service failures. It provides a way to monitor the availability and health of a service and automatically protect it from potential failures by “tripping” the circuit and preventing further requests from being sent to it.
Benefits of Circuit Breaker Pattern
- Fault Isolation: It isolates faulty services from the rest of the system, preventing cascading failures.
- Resilience: It makes the system more resilient to failures by allowing services to recover independently.
- Load Balancing: It helps distribute traffic more effectively by excluding failed services from the load balancer.
- Automatic Recovery: It automatically attempts to reconnect to the failed service when it becomes available again.
Essential Components of Circuit Breaker Pattern
- Circuit Breaker: This is the core component that monitors the health of the service and makes decisions about when to open or close the circuit.
- State: The Circuit Breaker maintains a state, typically Open, Half-Open, or Closed, based on the success or failure of recent requests.
- Health Monitoring: The Circuit Breaker continuously monitors the service’s health by sending periodic requests or listening for health checks.
- Failure Threshold: This is a predefined threshold that determines when the Circuit Breaker should trip and open the circuit.
- Timeout: It specifies the maximum time a request can take before being considered a failure.
- Retry Mechanism: When the circuit is in the Half-Open state, the Circuit Breaker allows a limited number of requests through to test if the service has recovered.
How the Circuit Breaker Pattern works
- Closed State: Initially, the Circuit Breaker is in the Closed state, allowing requests to flow to the service as usual.
- Open State: When the failure threshold is reached, the Circuit Breaker trips and enters the Open state. In this state, all requests are rejected, and no further requests are allowed to pass through.
- Half-Open State: After a timeout period, the Circuit Breaker transitions to the Half-Open state. In this state, it allows a limited number of requests through to check if the service has recovered. If these requests succeed, the Circuit Breaker transitions back to the Closed state. If they fail, the Circuit Breaker remains in the Open state for another timeout period.
Benefits of Circuit Breaker Pattern
- Improved Reliability: By handling failures gracefully, the Circuit Breaker pattern increases the reliability of the overall system.
- Reduced Latency: By preventing requests from being sent to failed services, the Circuit Breaker reduces the latency of successful requests.
- Increased Scalability: The Circuit Breaker pattern helps scale the system by allowing individual services to fail independently without impacting the entire system.
Recommended Practices for using the Circuit Breaker Pattern
- Configure Failure Thresholds Wisely: Set the failure threshold based on the expected failure rate and the impact of failures on the system.
- Tune Timeout Values: Adjust the timeout period to balance the need for quick failure detection with the potential for false positives.
- Use Retry Mechanisms Effectively: Limit the number of retries in the Half-Open state to prevent overloading the recovering service.
- Monitor Circuit Breaker Metrics: Keep track of Circuit Breaker metrics such as failure rates and open circuit durations to identify potential issues early. Conclusion:
Event Sourcing Pattern
Event sourcing involves capturing changes to the state of a system as a series of events. These events are stored in a persistent event store, which can be used to reconstruct the state of the system at any point in time.
Benefits of Event Sourcing
- Immutability: Events are immutable, which means that they cannot be changed once they have been created. This ensures that the history of the system is always accurate.
- Replayability: Events can be replayed in order to reconstruct the state of the system at any point in time. This is useful for debugging, disaster recovery, and testing.
- Scalability: Event sourcing can be used to scale a system horizontally by partitioning the event store. This allows multiple instances of the system to read and write events concurrently.
- Decoupling: Event sourcing can be used to decouple different components of a system. This makes it easier to develop and maintain the system, and it also improves resilience.
Drawbacks of Event Sourcing
- Complexity: Event sourcing can be more complex to implement than traditional data storage techniques.
- Performance: Event sourcing can be less performant than traditional data storage techniques, especially for queries that require access to a large number of events.
- Cost: Event sourcing can be more expensive to implement than traditional data storage techniques, especially if the event store is hosted in the cloud.
When to use Event Sourcing
Event sourcing is a good choice for systems that need to be:
- Immutable: The state of the system should not be able to be changed once it has been created.
- Replayable: The state of the system should be able to be reconstructed at any point in time.
- Scalable: The system needs to be able to handle a large number of events concurrently.
- Decoupled: The system needs to be able to be developed and maintained independently.
Proxy Design Pattern
The Proxy design pattern is a structural design pattern that provides a surrogate or placeholder for another object. The proxy controls access to the real object, allowing you to perform additional operations or security checks before granting access.
In the context of microservices, the Proxy design pattern can be used to:
-
Service Discovery and Load Balancing: A proxy can be used to discover available microservices and balance the load among them. This can help improve the performance and scalability of your microservices architecture.
-
Access Control and Security: A proxy can be used to enforce access control and security policies. It can verify the identity of the client and determine if they have the necessary permissions to access the real service.
-
Fault Tolerance and Resilience: A proxy can be used to implement fault tolerance and resilience mechanisms. It can handle failures of downstream microservices and retry requests or failover to alternative services.
-
Service Composition and Aggregation: A proxy can be used to compose and aggregate services. It can combine the functionality of multiple microservices into a single, cohesive interface.
-
Monitoring and Observability: A proxy can be used to monitor and observe the behavior of microservices. It can collect metrics and logs, which can be used to identify performance bottlenecks, errors, and potential security issues.
Here’s a simple example of how the Proxy design pattern can be implemented in a microservices architecture:
-
Create a proxy class that implements the same interface as the real service.
-
In the proxy class, add additional functionality or security checks.
-
Register the proxy class with the service registry.
-
When a client wants to access the service, it sends a request to the proxy.
-
The proxy class intercepts the request and performs the additional functionality or security checks.
-
If the checks are successful, the proxy class forwards the request to the real service.
-
The real service processes the request and returns a response.
-
The proxy class receives the response and forwards it to the client.
By using the Proxy design pattern, you can achieve better service discovery, load balancing, access control, fault tolerance, and monitoring in your microservices architecture. This can lead to improved performance, scalability, and reliability.
Chain of Responsibility Pattern
The Chain of Responsibility pattern allows a request to be passed along a chain of objects until one of them handles it. This pattern is useful for decoupling the sender of a request from the receiver, and it allows for the creation of modular, reusable components.
In a microservices architecture, the Chain of Responsibility pattern can be used to implement a request routing mechanism. In this scenario, there would be a series of microservices, each of which would be responsible for handling a specific type of request. When a request is received, it would be passed along the chain of microservices until one of them is able to handle it.
The Chain of Responsibility pattern can also be used to implement a service discovery mechanism. In this scenario, there would be a series of microservices, each of which would provide a specific type of service. When a client needs to use a particular service, it would send a request to a service discovery microservice. The service discovery microservice would then return a list of microservices that provide the requested service.
The Chain of Responsibility pattern is a powerful tool for designing microservices architectures. It can be used to decouple the sender of a request from the receiver, and it allows for the creation of modular, reusable components.
Here is an example of how the Chain of Responsibility pattern can be used in a microservices architecture:
- A client sends a request to a load balancer.
- The load balancer forwards the request to a service discovery microservice.
- The service discovery microservice returns a list of microservices that provide the requested service.
- The load balancer forwards the request to one of the microservices in the list.
- The microservice handles the request and returns a response.
- The load balancer forwards the response to the client.
In this example, the load balancer is responsible for forwarding the request to the appropriate microservice. The service discovery microservice is responsible for providing a list of microservices that provide the requested service. The microservices are responsible for handling the request and returning a response.
The Chain of Responsibility pattern allows for the creation of a modular, reusable architecture that can be easily scaled and maintained.
Shared Database Pattern
The Shared Database Pattern allows multiple microservices to share a single database. This pattern is typically used when the data is closely related and needs to be accessed by multiple services.
Benefits of Shared Database Pattern
- Reduced complexity: By sharing a single database, you can reduce the complexity of your system. You don’t have to worry about managing multiple databases, and you can ensure that all of your data is in sync.
- Improved performance: By sharing a single database, you can improve the performance of your system. This is because all of the data is stored in a single location, which makes it easier for the microservices to access it.
- Increased scalability: By sharing a single database, you can increase the scalability of your system. This is because you can add more microservices to your system without having to worry about adding more databases.
Challenges to using the Shared Database Pattern
- Increased coupling: By sharing a single database, you increase the coupling between your microservices. This means that if one microservice fails, it can impact the other microservices that are using the same database.
- Increased complexity: By sharing a single database, you increase the complexity of your system. This is because you have to ensure that all of the microservices are using the same data model and that the data is being accessed in a consistent manner.
- Increased risk of data loss: By sharing a single database, you increase the risk of data loss. This is because if the database fails, all of the data in the database will be lost.
Tips for using the Shared Database Pattern
- Use a single database for closely related data: The Shared Database Pattern is best suited for data that is closely related and needs to be accessed by multiple microservices. For example, you could use a shared database for customer data, product data, or order data.
- Use a data model that is compatible with all of the microservices: When you share a database between multiple microservices, it is important to use a data model that is compatible with all of the microservices. This will ensure that all of the microservices can access the data in the same way.
- Ensure that the data is being accessed in a consistent manner: When you share a database between multiple microservices, it is important to ensure that the data is being accessed in a consistent manner. This will help to prevent data corruption and ensure that all of the microservices are using the data in the same way.
- Monitor the database for performance issues: When you share a database between multiple microservices, it is important to monitor the database for performance issues. If the database is experiencing performance issues, it can impact the performance of all of the microservices that are using it.
CQRS Pattern
The CQRS pattern (Command Query Responsibility Segregation) is a software design pattern that separates the operations that modify data (commands) from the operations that read data (queries). This separation can improve the performance, scalability, and maintainability of a system.
In a microservices architecture, the CQRS pattern can be used to design microservices that are responsible for specific commands or queries. This can help to improve the modularity and isolation of the system, making it easier to develop and maintain.
Benefits of CQRS Pattern
- Improved performance: By separating commands and queries, you can optimize each type of operation for its specific needs. For example, you can use a NoSQL database for commands and a SQL database for queries.
- Increased scalability: By splitting the system into smaller, independent microservices, you can scale each microservice independently. This can help to improve the overall scalability of the system.
- Enhanced maintainability: By separating commands and queries, you can make it easier to understand and maintain the code. This can help to reduce the risk of errors and improve the overall quality of the system.
Here is an example of how the CQRS pattern can be used in a microservices architecture:
- Command microservice: This microservice is responsible for handling commands that modify data. For example, it might be responsible for creating, updating, or deleting products.
- Query microservice: This microservice is responsible for handling queries that read data. For example, it might be responsible for getting a list of all products or getting the details of a specific product.
By separating the commands and queries into two different microservices, you can improve the performance, scalability, and maintainability of the system.
Saga Pattern
The Saga pattern is a coordination pattern for distributed transactions. It is used to ensure that a series of related transactions are either all committed or all rolled back. This is achieved by using a series of compensating transactions that are executed in the reverse order of the original transactions.
The Saga pattern is often used in microservices architectures, where each microservice is responsible for a single transaction. This can make it difficult to ensure that all of the transactions in a series are completed successfully. The Saga pattern provides a way to coordinate these transactions and ensure that they are all either committed or rolled back.
The Saga pattern works as follows:
- A transaction is started in the first microservice.
- The first microservice calls the second microservice and passes it a message.
- The second microservice executes the transaction and sends a message back to the first microservice.
- The first microservice commits the transaction.
- If the second microservice fails, the first microservice executes a compensating transaction to roll back the transaction.
This pattern can be repeated for any number of microservices.
The Saga pattern has a number of advantages over other coordination patterns, such as two-phase commit and XA transactions. These advantages include:
- Simplicity: The Saga pattern is relatively simple to understand and implement.
- Flexibility: The Saga pattern can be used with any type of microservice.
- Scalability: The Saga pattern can be scaled to handle a large number of transactions.
However, the Saga pattern also has some disadvantages, such as:
- Latency: The Saga pattern can introduce latency into a system, as each transaction must wait for the previous transaction to complete.
- Complexity: The Saga pattern can become complex to manage, as the number of transactions increases.
- Performance: The Saga pattern can impact performance, as each transaction must be executed multiple times.
Overall, the Saga pattern is a powerful coordination pattern that can be used to ensure that a series of related transactions are either all committed or all rolled back. It is a good choice for microservices architectures, where each microservice is responsible for a single transaction.
Here are some examples of how the Saga pattern can be used in microservices architectures:
- Order processing: A customer places an order for a product on a website. The order is processed by a series of microservices, including a shopping cart service, a payment service, and a shipping service. The Saga pattern is used to ensure that all of the microservices involved in the order processing are either all successful or all rolled back.
- Inventory management: A company uses a microservice to track the inventory of its products. The microservice is updated whenever a product is purchased or sold. The Saga pattern is used to ensure that the microservice is updated correctly, even if there is a failure in the system.
- Customer relationship management: A company uses a microservice to track its customer relationships. The microservice is updated whenever a customer interacts with the company, such as when they make a purchase or contact customer support. The Saga pattern is used to ensure that the microservice is updated correctly, even if there is a failure in the system.
BFF Pattern
BFF (Backends For Frontends) is a microservices design pattern that creates a separate service for each frontend application. This allows the frontend and backend teams to work independently and deploy their code more frequently.
Benefits of BFF Pattern
- Improved performance: By having a separate service for each frontend application, the BFF can be tailored to the specific needs of that application. This can result in improved performance and responsiveness.
- Increased flexibility: The BFF pattern allows the frontend and backend teams to work independently. This means that the frontend team can make changes to the user interface without having to wait for the backend team to make changes to the backend code.
- Easier deployment: With the BFF pattern, each frontend application has its own service. This makes it easier to deploy new versions of the frontend application without having to redeploy the entire backend application.
Challenges of BFF Pattern
- Increased complexity: The BFF pattern can add complexity to your microservices architecture. You need to manage multiple services and ensure that they are all communicating properly.
- Increased cost: The BFF pattern can also increase your costs. You need to provision and manage multiple servers and you may need to purchase additional licenses for your software.
- Potential performance bottleneck: If the BFF is not properly designed, it can become a performance bottleneck. This is because the BFF is responsible for all communication between the frontend and backend applications.
Overall, the BFF pattern can be a useful tool for improving the performance, flexibility, and deployment of your microservices applications. However, it is important to understand the challenges of using this pattern before you decide to adopt it.
Strangler Pattern
Strangler Pattern is used for migrating a monolithic application to a microservices architecture without disrupting the existing system.
Concept
-
The goal of the Strangler Pattern is to gradually replace a monolithic application with a microservices architecture, one microservice at a time.
-
The monolithic application is wrapped or strangled by a layer of microservices that interact with the monolithic application through well-defined integration points.
-
As new microservices are added and integrated, the monolithic code is gradually decommissioned and replaced with microservices.
Steps to implement the Strangler Pattern
1. Identify Microservice Candidates
Identify the modules or components within the monolithic application that can be extracted as independent microservices.
2. Create Integration Points
- Define clear integration points between the monolithic application and the microservices.
- This can be done using REST APIs, message queues, or other communication mechanisms.
3. Develop Microservices
Develop the microservices one by one, starting with the ones that have the least dependencies on the monolithic application.
4. Interconnect Microservices
Integrate the microservices with each other and with the monolithic application through the defined integration points.
5. Route Traffic Gradually
- Gradually route traffic from the monolithic application to the microservices.
- This can be done in phases, starting with a small percentage of traffic and increasing it over time.
6. Monitor and Refactor
- Monitor the performance and stability of the microservices and the monolithic application.
- Refactor the code and improve the design of both the microservices and the monolithic application as needed.
7. Decommission Monolithic Code
- As more microservices are developed and integrated, the monolithic code becomes less used.
- Deprecate and eventually decommission the monolithic code as it is fully replaced by the microservices.
Benefits of Strangler Pattern
1. Gradual Migration
Allows for a gradual migration from a monolithic application to a microservices architecture, reducing the risk of disruption.
2. Minimized Downtime
By replacing parts of the monolithic application gradually, the risk of downtime during the migration is minimized.
3. Modularity and Flexibility
The microservices can be developed and managed independently, leading to increased modularity and flexibility.
4. Improved Scalability and Performance
The microservices architecture enables improved scalability and performance by allowing for horizontal scaling of individual services.
Considerations
1. Complexity
The Strangler Pattern can introduce additional complexity in terms of managing and coordinating multiple services and their integration points.
2. Testing and Monitoring
Thorough testing and monitoring are required to ensure that the integration between the monolithic application and the microservices functions properly.
3. Data Consistency
Managing data consistency across the monolithic application and the microservices can be a challenge that needs to be carefully addressed.
4. Expertise
Implementing the Strangler Pattern requires expertise in microservices design and development, as well as in integrating and managing distributed systems.
How to Choose Microservices Design Patterns
Understand Your System’s Requirements
- Identify the functional and non-functional requirements of your system.
- Consider factors such as scalability, availability, and security.
- Define clear boundaries and responsibilities for each microservice.
Select the Appropriate Architectural Style
- Choose a suitable architectural style, such as monolithic, distributed, or microservices.
- Consider the advantages and disadvantages of each style in the context of your system.
Identify Microservice Candidates
- Decompose your system into smaller, independent services.
- Look for natural boundaries, such as functional modules or business domains.
- Aim for services that are cohesive and loosely coupled.
Choose a Communication Mechanism
- Select a communication mechanism between microservices, such as HTTP, REST, gRPC, or message queues.
- Consider factors like latency, throughput, and reliability.
Define Service Contracts
- Establish clear and well-defined service contracts for each microservice.
- Specify the interface, data formats, and expected behavior of each service.
Implement Fault Tolerance and Resilience
- Design microservices to be fault-tolerant and resilient to failures.
- Incorporate mechanisms for handling errors, timeouts, and retries.
Consider Data Management
- Determine how data will be managed and shared among microservices.
- Choose a suitable data storage solution, such as a relational database, NoSQL database, or distributed cache.
Design for Scalability and Availability
- Ensure that microservices can scale horizontally to meet increasing demand.
- Implement mechanisms for load balancing and failover to improve availability.
Implement Service Discovery and Registration
- Implement a service discovery mechanism to allow microservices to find and communicate with each other.
- Consider using a service registry or a distributed DNS system.
Monitor and Observability
- Implement monitoring and observability tools to track the performance and health of your microservices.
- Use metrics, logs, and traces to identify issues and optimize your system.
Conclusion
Design patterns are a valuable tool for developers working on microservices architecture. By using proven, standardized solutions to common problems, developers can create more robust, maintainable, and scalable systems. The patterns mentioned above are just a few of the many design patterns that can be used in microservices architecture. By understanding and applying these patterns, developers can build systems that are better equipped to handle the complexities of distributed systems and meet the needs of modern software development.