RESTful API Best Practices
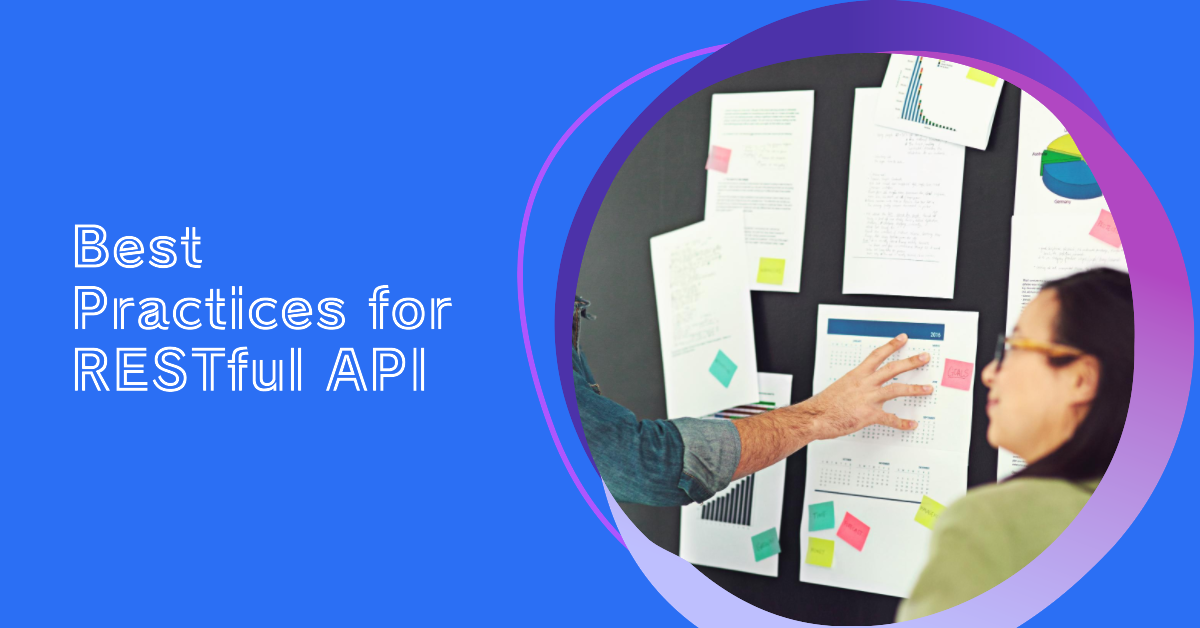
REST(Representational State Transfer) is an architectural style for designing networked applications. It is commonly used in web services development and provides a simple way to request and manipulate resources through HTTP protocol.
A RESTful API adheres to these constraints, making it easier to develop and manage APIs.
1. Use meaningful and consistent URLs
Use clear, concise words to describe your API endpoints. Use nouns (not verbs) and keep them in lower case.
One of the most important aspects of a RESTful API is the names of the resources being accessed. It’s crucial to use unique and meaningful names that accurately represent the resource being accessed, such as user/1234
or product/666
. This makes it easier for clients to understand what data they are accessing and reduces the risk of conflicts with other resources.
2. Use proper HTTP methods
HTTP request methods should be used to define the action being performed on a resource. Each method has a specific purpose and should not be interchanged.
GET
Retrieve data from a resource, e.g.:
- Fetch employee list
GET /api/v1/employee?limit=10&offset=0
- Fetch the specified employee information
GET /api/v1/employee/1
.
POST
Create a new resource, e.g., add a new employee:
|
|
PUT
Update an existing resource, e.g., update employee:
|
|
PATCH
Update a partial resource. It is similar to the PUT method, but instead of replacing the entire resource, it updates only the specified fields. e.g., update the email address for this employee but keep all other information intact:
|
|
DELETE
Remove a resource. e.g., delete an employee DELETE /api/v1/employee/1
.
HEAD
The HEAD
method asks for a response identical to a GET request, but without the response body.
Here’s an example of how to use the HEAD method:
Suppose you have a file named example.jpg
hosted on your server at the URL http://api.example.com/images/example.jpg
. Now, let’s say you want to check if this
image file exists and get some basic information about it (such as its size) without downloading the entire image data. To do this with a HEAD request, you would use
cURL (Command Line URL Request) like so:
|
|
In this example, the -I
option tells cURL to only show HTTP header information in its response. This will effectively prevent cURL from downloading the actual
content of the image file and instead provide you with the necessary metadata about it (such as its Content-Type, Content-Length, etc.).
Here’s an example of what the output might look like:
|
|
In this output, you can see that the HEAD request returned a 200 OK status code, indicating that the image file exists and is accessible. Additionally, it provides information about the content type (image/jpeg), content length (5857 bytes), last modified date, ETag, and more.
By using the HEAD method, you can efficiently retrieve metadata about resources in RESTful APIs without consuming unnecessary bandwidth by downloading their entire contents.
OPTIONS
The OPTIONS method describes the communication options for the target resource.
TRACE
The TRACE
method performs a message loop-back test along the path to the target resource.
3. Return appropriate HTTP status codes
HTTP status codes provide valuable information about the outcome of a request. Use them to convey the success or failure of your API calls.
1xx Informational responses
- 100 Continue
- 101 Switching Protocols
- 102 Processing
2xx Successful responses
- 200 OK
- 201 Created
- 202 Accepted
- 203 Non-Authoritative Information
- 204 No Content
- 205 Reset Content
- 206 Partial Content
- 207 Multi-Status
- 208 Already Reported
- 226 IM Used
3xx Redirection messages
- 300 Multiple Choices
- 301 Moved Permanently
- 302 Found
- 303 See Other
- 304 Not Modified
- 307 Temporary Redirect
- 308 Permanent Redirect
4xx Client error responses
- 400 Bad Request
- 401 Unauthorized
- 402 Payment Required
- 403 Forbidden
- 404 Not Found
- 405 Method Not Allowed
- 406 Not Acceptable
- 407 Proxy Authentication Required
- 408 Request Timeout
- 409 Conflict
- 410 Gone
- 411 Length Required
- 412 Precondition Failed
- 413 Payload Too Large
- 414 URI Too Long
- 415 Unsupported Media Type
- 416 Range Not Satisfiable
- 417 Expectation Failed
- 418 I’m a teapot
- 422 Unprocessable Entity
- 423 Locked
- 424 Failed Dependency
- 426 Upgrade Required
- 428 Precondition Required
- 429 Too Many Requests
- 431 Request Header Fields Too Large
- 451 Unavailable For Legal Reasons
5xx Server error responses
- 500 Internal Server Error
- 501 Not Implemented
- 502 Bad Gateway
- 503 Service Unavailable
- 504 Gateway Timeout
- 505 HTTP Version Not Supported
- 506 Variant Also Negotiates
- 507 Insufficient Storage
- 508 Loop Detected
- 510 Not Extended
- 511 Network Authentication Required
4. Validate inputs and handle errors gracefully
Input validation is essential for preventing malicious attacks or unintended data manipulation. Always validate user input before processing it. In case of any errors, provide meaningful error messages to help troubleshoot issues effectively.
- Validation: Check if the required fields are present in the request body and ensure that their values meet specific criteria (e.g., email format).
- Error handling: Catch exceptions and return appropriate error responses with clear descriptions of what went wrong.
|
|
5. Use pagination for large datasets
Pagination helps manage large amounts of data by dividing it into smaller, more manageable chunks. This improves performance and reduces the amount of information transferred over the network.
- Implement
?limit=10&offset=0
in your API to limit the number of records returned (e.g., 10) and set the starting point for those records (e.g., record 0).
6. Enable caching to improve performance
Caching reduces the number of requests made to your API by storing responses from previous calls. This improves performance and reduces server load.
- Set appropriate caching headers in your response (e.g.,
Cache-Control: max-age=600, public
). This will cache the response for 10 minutes (600 seconds) on clients that support caching.
7. Implement versioning for API updates
Versioning allows you to maintain backward compatibility while making changes to your API. This ensures that existing clients continue working even after new features are added or breaking changes are introduced.
- Include the API version in the URL (e.g.,
/api/v1/employee
). - Support multiple versions simultaneously, so clients can upgrade at their own pace.
8. Use JSON for data interchange
JSON (JavaScript Object Notation) is a lightweight and easy-to-parse format that works well with RESTful APIs. It’s widely supported by most programming languages and has lower overhead compared to XML.
- Respond with JSON data when fetching resources (e.g.,
{ "id": 1, "name": "John Doe" }
).
9. Secure Your API
- Use authentication mechanisms like OAuth, JWT etc.
- Use HTTPS.
- Use rate-limiting to prevent abuse.
10. Use If-Match and ETags Header for Concurrency Control
ETag (Entity Tag) is a unique identifier assigned to a resource in a RESTful API. It helps clients identify whether the resource has changed since their last request, which can be useful for conditional requests like PUT or DELETE.
To use ETags with a GET request, you should include the “If-Match” header in your HTTP request. This informs the server that you want to retrieve the resource only if its ETag matches the value provided in the header. If the resource has changed since your last request, the server will return a 412 Precondition Failed response instead of the requested data.
Here’s an example of how to use “If-Match” with a GET request:
|
|
In this example, the client is asking for the employee with ID 1 only if its ETag matches the value “e9c56f7d2324f08a941b26b9e3e9bf”. If the resource has been modified since the last request (i.e., its ETag no longer matches the provided value), the server will respond with a 412 Precondition Failed status code and not return the requested data.
This technique can help prevent race conditions when multiple clients are attempting to update the same resource simultaneously. By checking the ETag before performing an update, you can ensure that your changes don’t overwrite those made by another client in the meantime.
11. Implement API Documentation
API documentation is crucial for helping developers understand how to use your RESTful API effectively.
Use OpenAPI Specification (Swagger)
The OpenAPI Specification is a widely-adopted standard for describing REST APIs. By using this format, you can generate machine-readable and human-friendly documentation that includes detailed information about your API’s resources, endpoints, HTTP methods, request parameters, response data structures, etc.
Organize Your Documentation
Organize your API documentation into logical sections such as Overview, Authentication, Resources, Endpoints, etc., to make it easy for developers to find the information they need quickly.
Automatically generate code from OpenAPI documentation
OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3)
12. Continuously Monitor and Improve the API
Finally, it’s crucial to continuously monitor and improve the RESTful API over time. Use appropriate monitoring tools (such as metrics or log analysis) to identify areas for improvement and make changes accordingly. This helps ensure that the API remains scalable, secure, and easy to use over time.
By following these best practices, you can create a more efficient, reliable, and maintainable RESTful API that provides an excellent experience for your users.