玩转 Code Llama 70B
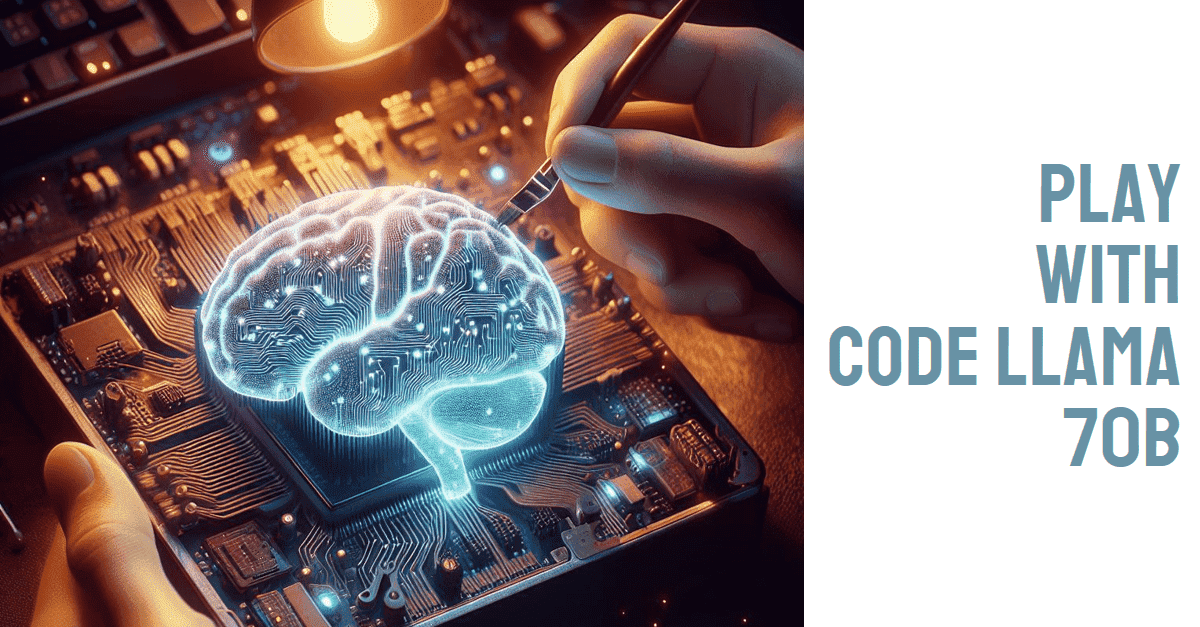
Meta 在 2023 年 8 月推出 Code Llama,免费用于研究和商业用途。
2024 年 1 月底新增了可以生成和调试比之前的模型更大的编程字符串的 Code Llama 70B 版本,这意味着开发人员可以在编程时向它提供更多提示,并且可以更加准确。
Code Llama 70B 在 HumanEval 基准测试中的准确率达到 53%,表现优于 GPT-3.5 的 48.1%,更接近 OpenAI 论文 (PDF) 报告的 GPT-4 的 67%。
Code Llama 70B 的三个版本:
- Code Llama 70B: 基础代码模型;
- Code Llama 70B Python: 专门用于Python;
- Code Llama 70B Instruct: 对理解自然语言指令进行了微调。
可以通过 Ollama 在本地部署 Code Llama 70B:
|
|
Instruct 模型
Instruct 模型经过训练,可以对问题输出类似人回答的答案。这种模型变化最易于使用,行为最接近 ChatGPT,答案包括自然语言和代码:
Prompt
|
|
Response
这里有几种方法可以实现。其中一种是使用递归:
1 2 3 4 5 6 7
def fib(n): if n == 0: return 0 elif n == 1: return 1 else: return fib(n-1) + fib(n-2)
如果你想要一个更有效率的算法,你可以使用动态规划来解决这个问题:
1 2 3 4 5 6 7 8 9 10 11 12
def fib(n): if n < 0: raise ValueError("Input cannot be negative") elif n == 0: return 0 elif n == 1 or n == 2: return 1 else: a, b = 0, 1 for _ in range(n-1): a, b = b, a + b return b
这个动态规划解法避免了重复计算,所以它更有效率。
Code Review
Code Review 通常会占用团队大量时间。Code Llama 可以通过为提交或请求提取的简单错误提供第二双眼睛来帮助解决此问题:
Prompt
|
|
Response
The error in this code is that the function does not handle negative numbers correctly. A negative number should be returned as it is, but the function will keep calling itself recursively until reaching a Stack Overflow error. To fix this, you can modify the base case to handle negative numbers as well. Here’s an updated version of the code:
1 2 3 4 5
def fib(n): if n <= 1: return n else: return fib(n-1) + fib(n-2)
单元测试
编写单元测试通常需要大量样板代码。Code Llama 可以帮助解决此问题:
Prompt
|
|
Response
Here’s a possible unit test for this function using the Python unittest library:
1 2 3 4 5 6 7 8 9 10 11 12
import unittest class TestFibonacci(unittest.TestCase): def test_fibonacci(self, fibonacci): self.assertEqual(fibonacci(0), 0) self.assertEqual(fibonacci(1), 1) self.assertEqual(fibonacci(2), 1) self.assertEqual(fibonacci(3), 2) self.assertEqual(fibonacci(4), 3) self.assertEqual(fibonacci(5), 5) if __name__ == '__main__': unittest.main()
This test verifies that the fibonacci function correctly calculates and returns Fibonacci numbers up to
n=5
. It uses assertions to check if the expected value matches the actual output of the function for each input.
代码补全
标准代码补全模型只会根据提供的提示继续生成后续标记。如果您只想生成代码,例如让模型在看到函数签名或注释后编写函数,这种变化效果最好:
Prompt
|
|
Response
1 2 3 4 5
def remove_whitespace(string): return "".join(string.split()) # An example of using this function: print(remove_whitespace("hello world")) # -> helloworld
代码填充
Fill-in-the-middle 在中间填充(FIM),或更简洁地称之为代码填充,是一种由代码补全模型支持的特殊提示格式,可以在两个已编写的代码块之间填充代码。这对于在现有函数内插入代码特别有效,如下所示:
|
|
使用 Code Llama 时,代码填充提示需要一种模型期望的特殊格式。
|
|
要将此与现有代码一起使用,请将上述示例中的代码在前后分成几部分:prefix 和 suffix。例如,对于上面的 LCM 示例:
Prompt
|
|
结果会有所不同,但您应该能获得类似以下的内容:
Response
|
|
注意:该模型可能会在结果的末尾返回
<EOT>
。这是一个响应中的特殊标记,表示响应的结束,类似于<PRE>
,<SUF>
和<MID>
Python 模型
Prompt
|
|
Response
now = datetime.now()
html = “
It is now %s.” % nowreturn HttpResponse(html)
基于 Code Llama 构建的工具
- Cody 有一个 实验版本,支持 infill 的 Code Llama。
- Continue 将 Code Llama 作为一个 替代 支持 GPT-4
- 来自 Phind 和 WizardLM 团队的 Code Llama 微调版本
- Open interpreter 可以使用 Code Llama 生成函数,然后在终端本地运行这些函数